RUBIX pipeline in steps#
RUBIX
is designed as a linear pipeline, where the individual functions are called and constructed as a pipeline. This allows as to execude the whole data transformation from a cosmological hydrodynamical simulation of a galaxy to an IFU cube in two lines of code. To get a better sense, what is happening during the execution of the pipeline, this notebook splits the pipeline in small steps.
This notebook contains the functions that are called inside the rubix pipeline. To see, how the pipeline is execuded, we refer to the notebook rubix_pipeline_single_function.ipynb
.
Step 1: Config#
The config
contains all the information needed to run the pipeline. Those are run specfic configurations. Currently we just support Illustris as simulation, but extensions to other simulations (e.g. NIHAO) are planned.
For the config
you can choose the following options:
pipeline
: you specify the name of the pipeline that is stored in the yaml file in rubix/config/pipeline_config.ymllogger
: RUBIX has implemented a logger to report the user, what is happening during the pipeline execution and give warningsdata - args - particle_type
: load only stars particle (“particle_type”: [“stars”]) or only gas particle (“particle_type”: [“gas”]) or both (“particle_type”: [“stars”,”gas”])data - args - simulation
: choose the Illustris simulation (e.g. “simulation”: “TNG50-1”)data - args - snapshot
: which time step of the simulation (99 for present day)data - args - save_data_path
: set the path to save the downloaded Illustris datadata - load_galaxy_args - id
: define, which Illustris galaxy is downloadeddata - load_galaxy_args - reuse
: if True, if in th esave_data_path directory a file for this galaxy id already exists, the downloading is skipped and the preexisting file is useddata - subset
: only a defined number of stars/gas particles is used and stored for the pipeline. This may be helpful for quick testingsimulation - name
: currently only IllustrisTNG is supportedsimulation - args - path
: where the data is stored and how the file will be namedoutput_path
: where the hdf5 file is stored, which is then the input to the RUBIX pipelinetelescope - name
: define the telescope instrument that is observing the simulation. Some telescopes are predefined, e.g. MUSE. If your instrument does not exist predefined, you can easily define your instrument in rubix/telescope/telescopes.yamltelescope - psf
: define the point spread function that is applied to the mock datatelescope - lsf
: define the line spread function that is applied to the mock datatelescope - noise
: define the noise that is applied to the mock datacosmology
: specify the cosmology you want to use, standard for RUBIX is “PLANCK15”galaxy - dist_z
: specify at which redshift the mock-galaxy is observedgalaxy - rotation
: specify the orientation of the galaxy. You can set the types edge-on or face-on or specify the angles alpha, beta and gamma as rotations around x-, y- and z-axisssp - template
: specify the simple stellar population lookup template to get the stellar spectrum for each stars particle. In RUBIX frequently “BruzualCharlot2003” is used.
# NBVAL_SKIP
import os
config = {
"pipeline":{"name": "calc_ifu"},
"logger": {
"log_level": "DEBUG",
"log_file_path": None,
"format": "%(asctime)s - %(name)s - %(levelname)s - %(message)s",
},
"data": {
"name": "IllustrisAPI",
"args": {
"api_key": os.environ.get("ILLUSTRIS_API_KEY"),
"particle_type": ["stars"],
"simulation": "TNG50-1",
"snapshot": 99,
"save_data_path": "data",
},
"load_galaxy_args": {
"id": 12,
"reuse": True,
},
"subset": {
"use_subset": True,
"subset_size": 1000,
},
},
"simulation": {
"name": "IllustrisTNG",
"args": {
"path": "data/galaxy-id-12.hdf5",
},
},
"output_path": "output",
"telescope":
{"name": "MUSE",
"psf": {"name": "gaussian", "size": 5, "sigma": 0.6},
"lsf": {"sigma": 0.5},
"noise": {"signal_to_noise": 1,"noise_distribution": "normal"},},
"cosmology":
{"name": "PLANCK15"},
"galaxy":
{"dist_z": 0.1,
"rotation": {"type": "edge-on"},
},
"ssp": {
"template": {
"name": "BruzualCharlot2003"
},
},
}
Step 2: RUBIX data format#
First, we have to download the simulation data from the Illustris webpage and store it and transform it to the rubixdata
format. The rubixdata
format is a unige format for the pipeline
. Any simulated galaxy can be transformed in the rubixdata
format, which enables RUBIX
to deal with all kind of cosmological hydrodynamical simulations of galaxies. For more deatails of this step, please have a look in the notebook create_rubix_data.ipynb
.
# NBVAL_SKIP
from rubix.core.data import convert_to_rubix, prepare_input
convert_to_rubix(config) # Convert the config to rubix format and store in output_path folder
rubixdata = prepare_input(config) # Prepare the input for the pipeline
2024-12-04 12:39:35,353 - rubix - INFO -
___ __ _____ _____ __
/ _ \/ / / / _ )/ _/ |/_/
/ , _/ /_/ / _ |/ /_> <
/_/|_|\____/____/___/_/|_|
2024-12-04 12:39:35,354 - rubix - INFO - Rubix version: 0.0.post138+g67c52e2.d20241203
2024-12-04 12:39:35,355 - rubix - INFO - Rubix galaxy file already exists, skipping conversion
2024-12-04 12:39:35,549 - rubix - INFO - Centering stars particles
2024-12-04 12:39:36,137 - rubix - WARNING - The Subset value is set in config. Using only subset of size 1000 for stars
You can simply access the data of the galaxy, e.g. the stellar coordinates by rubixdata.stars.coords
.
# NBVAL_SKIP
import matplotlib.pyplot as plt
# Make a scatter plot of the stars coordinates
plt.scatter(rubixdata.stars.coords[:,0], rubixdata.stars.coords[:,1], s=1)
<matplotlib.collections.PathCollection at 0x7f6097e4b640>
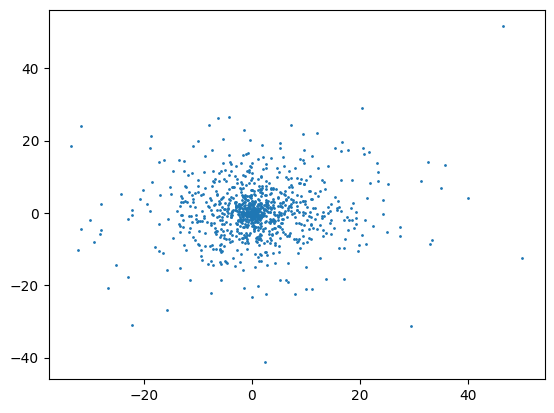
Step 3: Rotation#
In the config
we specify, how the galaxy should be orientated. In this example we want to orientate the galaxy edge-on
. We plot the coordinates again and see that they are now rotated.
# NBVAL_SKIP
from rubix.core.rotation import get_galaxy_rotation
rotate = get_galaxy_rotation(config)
rubixdata = rotate(rubixdata)
2024-12-04 12:39:36,772 - rubix - DEBUG - Roataion Type found: edge-on
2024-12-04 12:39:36,773 - rubix - INFO - Rotating galaxy with alpha=90.0, beta=0.0, gamma=0.0
# Make a scatter plot of the stars coordinates after rotation
plt.scatter(rubixdata.stars.coords[:,0], rubixdata.stars.coords[:,1], s=1)
<matplotlib.collections.PathCollection at 0x7f607475b160>
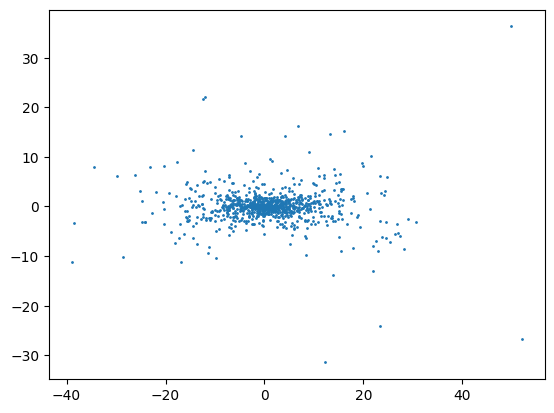
Step 4: Filter particles#
All particles outside field of view of the telescope are filtered. This has to be done, because we later bin the particles to the IFU grid and particles outside the arperture would make strange artefacts.
# NBVAL_SKIP
from rubix.core.telescope import get_filter_particles
filter_particles = get_filter_particles(config)
rubixdata = filter_particles(rubixdata)
2024-12-04 12:39:37,958 - rubix - INFO - Calculating spatial bin edges...
/home/annalena/rubix/rubix/telescope/factory.py:24: UserWarning: No telescope config provided, using default stored in /home/annalena/rubix/rubix/telescope/telescopes.yaml
warnings.warn(
2024-12-04 12:39:38,080 - rubix - INFO - Getting cosmology...
2024-12-04 12:39:38,315 - rubix - INFO - Filtering particles outside the aperture...
Step 5: Spaxel assignment#
We have an telescope aperture and a spatial resolution, which results in a spatial grid for the IFU cube. We can now assign the stars particles to the different spaxels in the IFU cube, i.e. define to which spaxel the stellar light of each stars particle contribute.
# NBVAL_SKIP
from rubix.core.telescope import get_spaxel_assignment
bin_particles = get_spaxel_assignment(config)
rubixdata = bin_particles(rubixdata)
/home/annalena/rubix/rubix/telescope/factory.py:24: UserWarning: No telescope config provided, using default stored in /home/annalena/rubix/rubix/telescope/telescopes.yaml
warnings.warn(
2024-12-04 12:39:38,438 - rubix - INFO - Calculating spatial bin edges...
/home/annalena/rubix/rubix/telescope/factory.py:24: UserWarning: No telescope config provided, using default stored in /home/annalena/rubix/rubix/telescope/telescopes.yaml
warnings.warn(
2024-12-04 12:39:38,450 - rubix - INFO - Getting cosmology...
2024-12-04 12:39:38,451 - rubix - INFO - Assigning particles to spaxels...
Step 6: Reshape data#
At the moment we have to reshape the rubix data that we can split the data on multiple GPUs. We plan to move from pmap to shard_map. Then this step should not be necessary any more. This step has purely computational reason and no physics motivated reason.
# NBVAL_SKIP
from rubix.core.data import get_reshape_data
reshape_data = get_reshape_data(config)
rubixdata = reshape_data(rubixdata)
Step 7: Spectra calculation#
This is the heart of the pipeline
. Now we do the lookup for the spectrum for each stellar particle. For the simple stellar population model by BruzualCharlot2003
, each stellar particle gets a spectrum assigned based on its age and metallicity. In the plot we can see that the spectrum differs for different stellar particles.
# NBVAL_SKIP
from rubix.core.ifu import get_calculate_spectra
calcultae_spectra = get_calculate_spectra(config)
rubixdata = calcultae_spectra(rubixdata)
2024-12-04 12:39:38,713 - rubix - WARNING - python-fsps is not installed. Please install it to use this function. Install using pip install fsps and check the installation page: https://dfm.io/python-fsps/current/installation/ for more details. Especially, make sure to set all necessary environment variables.
2024-12-04 12:39:38,868 - rubix - DEBUG - Method not defined, using default method: cubic
2024-12-04 12:39:38,869 - rubix - INFO - Calculating IFU cube...
2024-12-04 12:39:38,870 - rubix - DEBUG - Input shapes: Metallicity: 1, Age: 1
2024-12-04 12:39:39,833 - rubix - DEBUG - Calculation Finished! Spectra shape: (1, 1000, 842)
# NBVAL_SKIP
import jax.numpy as jnp
plt.plot(jnp.arange(len(rubixdata.stars.spectra[0][0][:])), rubixdata.stars.spectra[0][0][:])
plt.plot(jnp.arange(len(rubixdata.stars.spectra[0][0][:])), rubixdata.stars.spectra[0][1][:])
[<matplotlib.lines.Line2D at 0x7f60977bae60>]
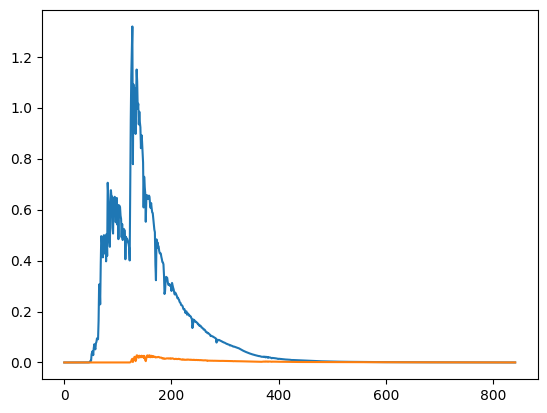
Step 8: Scaling by mass#
The stellar spectra have to be scaled by the stellar mass. Later heavier stellar particles should contribute more to the spectrum in a spaxel than lighter stellar particles.
# NBVAL_SKIP
from rubix.core.ifu import get_scale_spectrum_by_mass
scale_spectrum_by_mass = get_scale_spectrum_by_mass(config)
rubixdata = scale_spectrum_by_mass(rubixdata)
2024-12-04 12:39:40,261 - rubix - INFO - Scaling Spectra by Mass...
Step 9: Doppler shifting and resampling#
The stellar particles are not at rest and therefore the emitted light is doppler shifted with respect to the observer. Before adding all stellar spectra in each spaxel, we dopplershift the spectra according to their particle velocity and we resample the spectra to the wavelength grid of the observing instrument.
# NBVAL_SKIP
from rubix.core.ifu import get_doppler_shift_and_resampling
doppler_shift_and_resampling = get_doppler_shift_and_resampling(config)
rubixdata = doppler_shift_and_resampling(rubixdata)
/home/annalena/rubix/rubix/telescope/factory.py:24: UserWarning: No telescope config provided, using default stored in /home/annalena/rubix/rubix/telescope/telescopes.yaml
warnings.warn(
2024-12-04 12:39:40,379 - rubix - DEBUG - SSP Wave: (842,)
2024-12-04 12:39:40,480 - rubix - INFO - Doppler shifting and resampling stellar spectra...
2024-12-04 12:39:40,481 - rubix - DEBUG - Doppler Shifted SSP Wave: (1, 1000, 842)
2024-12-04 12:39:40,481 - rubix - DEBUG - Telescope Wave Seq: (3721,)
# NBVAL_SKIP
from rubix.core.pipeline import RubixPipeline
pipe = RubixPipeline(config)
wave = pipe.telescope.wave_seq
print(wave)
print(rubixdata.stars.spectra[0][0][:])
plt.plot(wave, rubixdata.stars.spectra[0][0][:])
plt.plot(wave, rubixdata.stars.spectra[0][1][:])
/home/annalena/rubix/rubix/telescope/factory.py:24: UserWarning: No telescope config provided, using default stored in /home/annalena/rubix/rubix/telescope/telescopes.yaml
warnings.warn(
2024-12-04 12:39:41,167 - rubix - INFO - Getting rubix data...
2024-12-04 12:39:41,168 - rubix - INFO - Rubix galaxy file already exists, skipping conversion
2024-12-04 12:39:41,227 - rubix - INFO - Centering stars particles
2024-12-04 12:39:41,537 - rubix - WARNING - The Subset value is set in config. Using only subset of size 1000 for stars
2024-12-04 12:39:41,546 - rubix - INFO - Data loaded with 1000 star particles and 0 gas particles.
2024-12-04 12:39:41,547 - rubix - INFO - Data loaded with 48 properties.
2024-12-04 12:39:41,548 - rubix - DEBUG - Data: RubixData(galaxy=Galaxy(redshift=2.220446049250313e-16, center=array([11413.86337268, 35893.86374042, 32027.01940138]), halfmassrad_stars=7.727193253526112), stars=StarsData(coords=Array([[ 0.01953125, 0.12109375, 0.1953125 ],
[ 3.305664 , 1.5234375 , -1.8144531 ],
[ 1.4316406 , 5.6953125 , -1.4589844 ],
...,
[-0.22949219, -0.0546875 , -0.02148438],
[15.157227 , -4.2460938 , 4.4746094 ],
[14.027344 , -0.015625 , -2.1054688 ]], dtype=float32), velocity=Array([[ 2.2396517e-15, 2.4131075e-15, -4.0351599e-15],
[ 9.0712782e-17, 8.7445301e-15, -6.1377769e-15],
[-4.2026344e-15, 1.9314719e-15, 7.7893150e-17],
...,
[ 5.8114825e-16, -1.3959399e-16, 3.1129105e-15],
[ 8.0419468e-16, 2.5377044e-15, -2.7024722e-15],
[-2.7399760e-15, 2.3714432e-15, -2.5337364e-15]], dtype=float32), mass=Array([103706.484, 73743.086, 70998.35 , 85374.91 , 82220.21 ,
88501.25 , 138703.16 , 98413.58 , 114730.28 , 110815.65 ,
106835.34 , 82952.766, 55514.266, 45093.457, 119139.87 ,
99465.29 , 81894.88 , 99098.49 , 121604.28 , 73959.84 ,
81568.74 , 77949.09 , 63735.67 , 83705.78 , 88831.94 ,
94049.12 , 128544.5 , 111424.14 , 85921.4 , 81257.164,
120619.51 , 91774.32 , 146891.14 , 112913.516, 86486.46 ,
95861.19 , 106234.39 , 99594.17 , 84475.86 , 88601.766,
123305.914, 92989.03 , 80892.92 , 116025.11 , 92752.86 ,
133442.92 , 58536.78 , 75977.53 , 107455.69 , 102766.82 ,
69256.67 , 90629.86 , 113887.055, 94529.17 , 98055.66 ,
145879.19 , 79203.086, 60170.086, 101019.29 , 96633.11 ,
89888.5 , 119878.25 , 106734.414, 71238.766, 107306.516,
52672.63 , 93005.36 , 95320.91 , 52186.527, 130619.73 ,
70907.23 , 83238.39 , 60344.555, 126573.48 , 119303.85 ,
106244.78 , 117947.8 , 90023.53 , 130132.05 , 154391.06 ,
108217.01 , 108079.766, 70043.76 , 97929.53 , 135604.33 ,
100191.43 , 141311.58 , 66223.63 , 77482.83 , 117216.625,
94993.87 , 81198.43 , 103967.98 , 119972.016, 63966.664,
84706.984, 93145.51 , 91789.97 , 138873.17 , 97771.766,
78426.984, 82272.12 , 95569.23 , 111268.99 , 69179. ,
69945.21 , 93762.21 , 77290.44 , 99038.3 , 102819.836,
121096.664, 86104.16 , 115770.76 , 71230.914, 102111.65 ,
127704.99 , 100001.27 , 122007.21 , 98949.87 , 108799.62 ,
45367.477, 87785.29 , 85747.45 , 91871.68 , 129286.016,
157948.77 , 99121.81 , 65377.97 , 76733.04 , 98953.19 ,
143347.9 , 85568.734, 114235.586, 116849.25 , 70448.96 ,
79609.516, 82454.75 , 76978.05 , 72914.914, 82279.41 ,
108404.42 , 102680.914, 75665.92 , 95232.195, 104924.44 ,
161324.4 , 93109.43 , 106213.39 , 69224.35 , 91687.19 ,
94972.766, 100404.36 , 64690.22 , 108578.83 , 96024.46 ,
87660.586, 71993.32 , 92670.36 , 118846.34 , 88499.09 ,
121490.41 , 106298.35 , 87116.16 , 84743.04 , 141193.3 ,
150384.14 , 94293.445, 80642.33 , 75813.09 , 80358.47 ,
90295.125, 67994.05 , 89223.81 , 131639.22 , 92760.51 ,
109354.41 , 56792.426, 57390.582, 90733.32 , 90823.99 ,
76613.03 , 133907.92 , 72256.62 , 99349.07 , 121417.46 ,
113764.77 , 61972.594, 87527.555, 74782.195, 79731.51 ,
118405.48 , 86716.1 , 73361.21 , 95322.21 , 80881.914,
125435.164, 136370.03 , 55025.703, 95667.33 , 115835.6 ,
90640.85 , 105854.73 , 131222.5 , 80385.945, 82782.75 ,
71727.4 , 58484.926, 85653.836, 75960.25 , 93423.33 ,
88612.37 , 91737.984, 88118.95 , 128495.055, 62555.863,
84572.78 , 68407.47 , 101724.195, 92262.75 , 90828.14 ,
102337.19 , 105714.914, 70140.5 , 87052.44 , 87405.01 ,
103563.17 , 117247.99 , 112633.99 , 76865.91 , 109124.72 ,
94626.97 , 61512.06 , 161041.08 , 112796.664, 116881.27 ,
88419.26 , 73282.375, 99186.67 , 90680.75 , 87184.65 ,
87714.12 , 107003.234, 122223.24 , 113636.85 , 71009.87 ,
119632.67 , 129031.82 , 97809.13 , 100149.53 , 133759.66 ,
72218.52 , 105218.48 , 91820.16 , 116302.01 , 120828.23 ,
66523.85 , 95621.99 , 121244.63 , 140439.47 , 86387.14 ,
92905.98 , 103331.92 , 73082.016, 80244.8 , 104475.71 ,
62483.504, 104800.89 , 90167.516, 128359.22 , 76597.09 ,
62095.586, 66116.41 , 122153.47 , 107697.555, 105410.26 ,
95080.22 , 72365.89 , 96533.89 , 90342.31 , 104238.81 ,
164011.89 , 73281.19 , 77675.83 , 103286.61 , 92131.945,
79492.234, 103175.414, 91831.54 , 74050.64 , 122732.36 ,
97924.805, 75816.555, 106413.65 , 89908.84 , 140560.66 ,
77965.2 , 121917.23 , 121019.54 , 89423.484, 83513.23 ,
96105.7 , 122074.25 , 86650.43 , 63591.887, 101602.74 ,
107806.39 , 108742.555, 94687.79 , 73712.37 , 89079.66 ,
79479.555, 62432.58 , 85436.15 , 139891.31 , 88966.83 ,
122616.48 , 64631.375, 92514.47 , 97164.49 , 113423.41 ,
45712.81 , 134149.28 , 78758.06 , 137630.05 , 146748.3 ,
107416.984, 70812.12 , 89469.23 , 64776.766, 108347.99 ,
93304.42 , 116929.016, 123408.125, 97897.84 , 88075.08 ,
77202.62 , 79226.24 , 86912.29 , 87327.734, 77936.13 ,
104750.51 , 105350.86 , 80376.09 , 77657.15 , 117382.95 ,
90714.26 , 86512.28 , 53954.14 , 95636.65 , 116473.32 ,
81456.1 , 103083.73 , 94223.055, 104848.16 , 70106.12 ,
120994.48 , 82119.76 , 116493.86 , 74859.914, 94340.836,
75720.14 , 75011.336, 129385.84 , 102488.25 , 94594.695,
114385.22 , 78330.77 , 112164.625, 107609.805, 118911.195,
61688.555, 110717.164, 110507.234, 89349.44 , 102691.125,
88979.84 , 103455.71 , 92368.15 , 95294.35 , 59448.61 ,
74083.42 , 100776.664, 66297.5 , 94908.48 , 91836.984,
93289.81 , 90402.59 , 80194.81 , 95950.65 , 89125.49 ,
134062. , 75205.12 , 98685.984, 71394.34 , 76059.44 ,
79033.55 , 69241.84 , 55896.543, 109183.26 , 58432.984,
83764.76 , 72993.99 , 124567.836, 93393.69 , 106451.43 ,
114471.18 , 63391.39 , 84105.04 , 82900.2 , 114317.4 ,
121177.24 , 95629.414, 80614.95 , 132495.34 , 143995.67 ,
57900.36 , 83696.82 , 135735.75 , 101862.63 , 59422.336,
79934.445, 79776.41 , 88711.46 , 94004.16 , 118422.9 ,
54291.27 , 106824.33 , 88080.96 , 83281.29 , 144200.1 ,
121297.48 , 92788.68 , 71587.86 , 71693.83 , 79290.7 ,
100440.28 , 110222.07 , 95124.12 , 91107.91 , 94596.56 ,
75741.375, 48096.395, 113035.07 , 99908.4 , 111697.62 ,
93423.266, 126490.25 , 73289.72 , 85496.56 , 100744.2 ,
100672.234, 84681.38 , 117332.234, 113048.16 , 89121.586,
128322.1 , 94701.75 , 95786.97 , 125852.85 , 80056.19 ,
122384.125, 102609.586, 101684.03 , 58844.95 , 97872.625,
102129.7 , 99610.26 , 112077.45 , 79936.3 , 95699.16 ,
94128.02 , 109341.73 , 70908.95 , 100342.234, 109257.484,
128694.734, 40169.094, 103509.96 , 50607.918, 98546.914,
78877.09 , 109361.26 , 101660.93 , 71001.63 , 89517.19 ,
113630.25 , 72683.11 , 95688.58 , 71497.89 , 71388.85 ,
78820.04 , 81286.6 , 62205.195, 110225.84 , 83703.58 ,
98517.15 , 60689.215, 80968.52 , 104963.695, 59065.91 ,
97302.87 , 86533.45 , 80444.84 , 89597.516, 69351.96 ,
103612.15 , 84449.63 , 86945.01 , 110613.78 , 91861.61 ,
129047.57 , 112316.64 , 131168.36 , 105665.69 , 83869.02 ,
98838.48 , 91249.914, 72762.31 , 92261.95 , 91180.35 ,
105055.83 , 99701.25 , 109368.98 , 94266.46 , 91842.75 ,
97174.195, 69864.81 , 111580.67 , 73000.48 , 116982.64 ,
97039.51 , 96735.54 , 82170.31 , 76772.4 , 71373.914,
93942.95 , 137930.89 , 73491.305, 45522.305, 72374.484,
98368.984, 69098.63 , 90592.67 , 107103.74 , 155540. ,
113036.85 , 138120.8 , 91674.29 , 90031.555, 63334.51 ,
124379.86 , 75419.94 , 74885.7 , 115947.67 , 81708.83 ,
144293.94 , 95200.266, 88370.79 , 144164.12 , 94730.836,
107308.83 , 167453.17 , 95462.266, 81414.38 , 98816.28 ,
117500.266, 106049.414, 142834.23 , 140332.02 , 55017.43 ,
108087.39 , 93460.63 , 116811.555, 79292.55 , 81632.055,
86589.23 , 94405.03 , 66433.164, 97646.76 , 86446.08 ,
104727.164, 139598.11 , 95858.75 , 101942.39 , 93054.94 ,
93679.945, 67439.805, 72444.99 , 110807.77 , 74849.98 ,
83353.97 , 100162.94 , 112900.91 , 102895.64 , 113664.266,
83197.42 , 63005.555, 90588.914, 92638.6 , 74182.07 ,
81099.38 , 102329.945, 72664.53 , 100871.68 , 117361.1 ,
101378.945, 101945.266, 97662.08 , 113930.7 , 76603.96 ,
101375.45 , 136521.72 , 104288.27 , 83660.7 , 60995.26 ,
69730.45 , 80142.25 , 103045.85 , 66783.56 , 119343.69 ,
94661.75 , 89706.875, 89695.37 , 84743.04 , 79419.92 ,
140119.45 , 129846.63 , 76032.55 , 103887.63 , 71698.29 ,
102096.18 , 90808.49 , 64946.12 , 127416.14 , 76151.29 ,
92710.81 , 79896.25 , 75339.48 , 112669.984, 93495.586,
90814.48 , 101308.23 , 118459.984, 119426.266, 83594.17 ,
57298.395, 114240.125, 86101.25 , 128737.82 , 74623.414,
114252.56 , 89449.62 , 78304.305, 79548.66 , 118591.83 ,
72985.625, 105386.86 , 111986.7 , 118763.41 , 93660.33 ,
87847.32 , 109689.42 , 76494.89 , 97502.62 , 130443.945,
86120.92 , 96781.445, 82427.7 , 81910.42 , 83105.836,
78988.875, 79368.38 , 89549.516, 72950.87 , 103732.28 ,
83353.055, 126053.445, 113373.66 , 91909.93 , 66809.305,
121739.266, 74398.3 , 99692.12 , 124474.56 , 102081.9 ,
119546.28 , 84958.47 , 106927.4 , 111892.76 , 112320.1 ,
110633.14 , 119503.48 , 59511.8 , 106983.055, 135738.12 ,
66386.984, 100819.52 , 110342.87 , 106715. , 59176.992,
86112.836, 126786.71 , 65583.85 , 103620.88 , 81640.16 ,
71154.37 , 116278.39 , 77073.414, 93145.48 , 139995.9 ,
84292.93 , 75677.74 , 85022.34 , 77055.58 , 78970.75 ,
106841.43 , 98551.36 , 77637.7 , 162873.17 , 58680.06 ,
74162.45 , 125901.73 , 93518.54 , 114768.2 , 98853.58 ,
98435.64 , 93291.266, 90789.625, 91608.21 , 98526.66 ,
88778.94 , 118339.29 , 88254.664, 114547.96 , 106083.414,
86156.58 , 96394.805, 125383.17 , 123755.484, 67516.75 ,
117885.08 , 93608.42 , 69339.62 , 97533.18 , 105075.2 ,
51938.926, 69717.9 , 147494.34 , 77910.3 , 121322.48 ,
61264.09 , 75327.64 , 128240.94 , 81286.87 , 90709.99 ,
67640.93 , 123451.73 , 68001.734, 122384.25 , 126877.87 ,
117985.664, 78287.3 , 123450.836, 110381.2 , 99409.75 ,
99479.65 , 103106.3 , 114135.875, 61217.176, 101965.65 ,
103414.09 , 135256.56 , 121449.45 , 57194.496, 91295.305,
102966.25 , 97068.51 , 98563.59 , 86400.22 , 106492.01 ,
101443.16 , 100645.34 , 94879.56 , 107999.74 , 73824.07 ,
64908.94 , 76843.07 , 95568.61 , 93093.64 , 102083.984,
61437.625, 68695.61 , 134152.98 , 68294.305, 51138.94 ,
150865.58 , 80437.695, 135526.67 , 122776. , 96554.89 ,
90112.58 , 74083.195, 127246.52 , 127033.06 , 96882.98 ,
80026.875, 80930.34 , 119916.57 , 85523.445, 99842.32 ,
83888.79 , 87155.62 , 92761.164, 89285.36 , 55887.156,
118178.26 , 84300.805, 61603.375, 79690.805, 101982.266,
127704.62 , 95493.33 , 96061. , 111350.79 , 48169.156,
97612.9 , 93948.13 , 83332.766, 80944.54 , 117019.625,
88740.27 , 102357.37 , 78393.75 , 115471.49 , 97645.01 ,
145121.5 , 66540.836, 124100.04 , 92683.49 , 92247.15 ,
111519.766, 87818.586, 99559.21 , 78815.93 , 105439.84 ,
110950.19 , 120310.42 , 105773.82 , 106410.71 , 84529.35 ,
107824.87 , 96241.516, 91291.43 , 104016.55 , 86593.66 ,
126362.52 , 156541.92 , 133678.94 , 74640.31 , 79126.53 ,
121217.89 , 108098.664, 87873.484, 115539.945, 80791.67 ,
61723.016, 102573.586, 101548.695, 66237.93 , 84449.63 ,
66494.016, 115187.12 , 82161.68 , 77564.26 , 110085.71 ,
104859.82 , 96234.55 , 89221.74 , 121338.97 , 58262.71 ,
110025.23 , 80679.61 , 78503.43 , 123528.52 , 86552.13 ,
90678.35 , 78640.84 , 167147.02 , 73780.76 , 94175.42 ,
118392.98 , 119783.14 , 80135.125, 104012.75 , 63262.258,
104551.086, 92350.83 , 104334.375, 90642.86 , 87326.836,
85779.14 , 62959.336, 82141.125, 130775.91 , 102592.1 ,
87474.766, 85142.87 , 102306.13 , 81084.805, 112683.664,
81552.51 , 93854.336, 96227.35 , 84903.47 , 91303.914,
82539.9 , 112662.05 , 118337.23 , 105488.93 , 148276.38 ,
81203.48 , 107575.43 , 76721.07 , 73429.87 , 104041.26 ,
82284.91 , 136075.72 , 83457.66 , 65489.133, 71895.59 ,
145136.44 , 78835.914, 86466.66 , 115032.26 , 64957.645,
59511.55 , 97112.25 , 124349.14 , 150269.53 , 93942.47 ,
100354.086, 87976.27 , 81958.59 , 88674.83 , 116495.89 ,
106912.72 , 119892.164, 66159.62 , 52966.676, 72642.04 ,
88403.59 , 93307.8 , 99242.52 , 98736.59 , 148040.95 ,
84276.25 , 96527.92 , 85049.39 , 80746.04 , 93362.44 ,
78670.55 , 107781.86 , 122784.875, 86016.07 , 75101.89 ,
107408.555, 66126.84 , 58988.523, 114953.21 , 99559.93 ,
111940.07 , 61000.266, 117808.82 , 50440.54 , 106986.67 ,
100381.875, 97415.086, 80488.54 , 91536.21 , 76705.63 ,
92234.57 , 113394.6 , 73693.625, 111728.21 , 83877.85 ,
88669.125, 97748.71 , 85886.38 , 99049.36 , 118472.5 ,
91514.836, 103692.016, 82229.164, 82161.4 , 119349.664,
122307.17 , 88993.94 , 107585.43 , 112269.72 , 81224.26 ], dtype=float32), metallicity=Array([2.01166738e-02, 1.97864678e-02, 2.31143460e-02, 5.25850104e-03,
1.55215757e-02, 3.87870101e-03, 1.41741121e-02, 6.58358308e-03,
1.05137350e-02, 1.71273910e-02, 7.45867053e-03, 2.56497413e-02,
2.10167114e-02, 3.91210765e-02, 3.76510695e-02, 6.23573363e-02,
1.23941470e-02, 6.22132374e-03, 9.69346613e-03, 6.47366270e-02,
1.05168968e-02, 3.62057751e-03, 6.15874957e-03, 1.08359670e-02,
6.65692799e-03, 3.14099006e-02, 5.62439533e-03, 8.13409127e-03,
5.15206391e-03, 2.33183056e-02, 2.52892114e-02, 1.02555612e-03,
2.03775484e-02, 1.04270494e-02, 7.67303072e-03, 3.12927063e-03,
3.35235484e-02, 1.79153215e-02, 1.26082916e-02, 2.94010155e-02,
5.76207526e-02, 2.81321835e-02, 9.97730531e-03, 3.77879590e-02,
6.98206061e-03, 3.54802087e-02, 1.19106732e-02, 5.16092731e-03,
8.30952637e-03, 5.99885453e-03, 1.96811045e-03, 6.35805074e-03,
2.51067653e-02, 3.58630042e-03, 5.51027479e-03, 5.29555278e-03,
2.16334201e-02, 9.28492844e-03, 4.59532952e-03, 1.09685048e-01,
2.43569203e-02, 3.27996127e-02, 2.99373679e-02, 1.37991300e-02,
1.44221475e-02, 1.64612178e-02, 4.13357373e-03, 2.36468134e-03,
3.98098771e-03, 2.70883180e-03, 2.76608057e-02, 4.56860196e-03,
1.64166023e-03, 7.45402798e-02, 5.00043575e-03, 2.26901546e-02,
2.22091191e-02, 2.50603594e-02, 1.58966575e-02, 5.46064414e-03,
5.83660491e-02, 6.24286570e-02, 1.42574245e-02, 5.83855659e-02,
2.92432471e-03, 3.74578987e-03, 1.54689364e-02, 1.26187876e-02,
4.25996399e-03, 1.26889367e-02, 2.73110513e-02, 2.09241789e-02,
4.34007235e-02, 9.53575131e-03, 2.07999367e-02, 8.55443813e-03,
3.73770036e-02, 1.93719212e-02, 5.48822135e-02, 1.25969080e-02,
8.12614337e-03, 1.66955274e-02, 2.68233637e-03, 2.54292297e-03,
6.29712734e-03, 1.19550584e-03, 8.70161206e-02, 1.35744298e-02,
1.09639093e-02, 5.20155858e-03, 9.22962464e-03, 1.98547449e-02,
1.02741398e-01, 3.54661397e-03, 8.26401543e-03, 4.44174139e-03,
5.39483912e-02, 1.61268469e-02, 2.55766809e-02, 8.07874277e-03,
2.60682381e-03, 1.94895938e-02, 2.95487400e-02, 1.84974577e-02,
5.60203847e-03, 6.30011084e-03, 6.43693283e-02, 8.37542582e-03,
7.76874274e-02, 1.77104156e-02, 4.80895601e-02, 1.10832555e-02,
1.97629016e-02, 6.61762059e-02, 3.99622694e-03, 9.11395345e-03,
1.20570399e-02, 1.72018856e-02, 1.63687505e-02, 2.32192446e-02,
2.23097019e-03, 4.06020042e-03, 2.49430332e-02, 1.95889287e-02,
1.12813003e-02, 1.05480487e-02, 5.83604947e-02, 1.08964145e-02,
1.27957491e-02, 5.66865038e-03, 1.82160269e-02, 4.69507463e-03,
1.66742597e-02, 2.66964920e-02, 1.03644822e-02, 6.18219562e-03,
5.93340117e-03, 8.22725371e-02, 1.97166074e-02, 3.12804221e-03,
3.19330692e-02, 1.83585193e-02, 6.08493527e-03, 2.31764745e-02,
3.42552178e-02, 3.19008119e-02, 5.21238372e-02, 1.62393413e-02,
2.79191099e-02, 1.72897018e-02, 2.29435656e-02, 5.04432246e-02,
2.37412155e-02, 3.92046943e-03, 5.54945804e-02, 7.48974606e-02,
1.33114448e-02, 6.83625191e-02, 1.02139758e-02, 2.71645300e-02,
7.55627379e-02, 2.11905167e-02, 3.85848507e-02, 2.66387127e-02,
4.32240814e-02, 8.36951286e-03, 1.45925572e-02, 1.80864129e-02,
2.93777455e-02, 2.45033801e-02, 1.95222795e-02, 8.10367521e-03,
1.67843904e-02, 1.95473619e-02, 2.12934762e-02, 1.34029109e-02,
5.05686272e-03, 9.32900086e-02, 3.50835733e-02, 6.55144127e-03,
9.27358586e-03, 8.75244942e-03, 2.13629548e-02, 1.71630010e-02,
1.88917741e-02, 1.71294678e-02, 3.02424678e-03, 2.63313204e-02,
1.03140920e-01, 9.55885276e-03, 1.38192251e-02, 3.98579836e-02,
8.22342336e-02, 6.67627528e-03, 1.66392568e-02, 1.83230489e-02,
2.20910478e-02, 1.46797737e-02, 3.71291079e-02, 1.01838946e-01,
1.13612525e-02, 1.17596807e-02, 1.74762346e-02, 2.65788455e-02,
2.51167696e-02, 7.54309213e-03, 1.80272979e-03, 2.44575683e-02,
1.18079036e-02, 1.44928591e-02, 1.67716518e-02, 1.51306763e-02,
2.71675922e-02, 2.16924474e-02, 7.84497056e-03, 1.31294392e-02,
4.94469628e-02, 4.29502986e-02, 6.25146478e-02, 1.01161217e-02,
2.94281244e-02, 1.74296480e-02, 2.36924794e-02, 1.51163787e-02,
3.50722820e-02, 1.21720694e-02, 1.93042811e-02, 3.65400538e-02,
3.08704879e-02, 7.04939477e-03, 1.29368519e-02, 7.18010636e-03,
1.78310983e-02, 2.22939812e-02, 1.26137948e-02, 1.47617292e-02,
1.70548540e-02, 4.12269942e-02, 2.14205217e-03, 5.89751936e-02,
1.72583796e-02, 1.20149963e-02, 1.78089067e-02, 3.39201442e-03,
3.35718542e-02, 4.21757484e-03, 7.37143261e-03, 3.08433175e-02,
5.77196851e-03, 1.13602886e-02, 6.29300401e-02, 7.46589079e-02,
2.64636204e-02, 1.57459006e-02, 1.73268113e-02, 8.65969900e-03,
4.31788079e-02, 8.62016925e-04, 1.35981329e-02, 2.06957888e-02,
4.19494975e-03, 1.43427141e-02, 2.69425027e-02, 4.21396084e-03,
5.66849485e-02, 2.43340991e-02, 8.26040283e-03, 1.67260729e-02,
5.10209091e-02, 3.96402590e-02, 1.30390171e-02, 6.50478620e-03,
1.79871898e-02, 1.22591825e-02, 9.69969705e-02, 1.23190861e-02,
9.01102927e-03, 9.48138069e-03, 2.53643468e-02, 2.46302057e-02,
1.06304418e-02, 1.34908240e-02, 3.76397138e-03, 7.74635142e-03,
4.79883468e-03, 2.20464431e-02, 3.25407158e-03, 1.56453382e-02,
5.94981760e-03, 3.81142180e-03, 1.05864359e-02, 3.07791885e-02,
7.79884309e-03, 1.27201406e-02, 1.26873255e-02, 4.57380340e-02,
1.69336330e-02, 8.48805346e-03, 3.06954961e-02, 1.11712161e-02,
1.53625403e-02, 5.62178269e-02, 2.75508631e-02, 3.63867893e-03,
4.04114611e-02, 6.93086442e-03, 8.12801812e-03, 1.38652707e-02,
2.92993966e-03, 7.52554685e-02, 2.18100008e-02, 2.94876960e-03,
2.31938120e-02, 1.92542262e-02, 1.07678361e-02, 6.75670383e-03,
2.46528964e-02, 1.54268550e-04, 1.13951443e-02, 7.14404806e-02,
3.51478420e-02, 6.66432735e-03, 1.21032260e-02, 1.77804120e-02,
2.07910072e-02, 1.61141474e-02, 8.12683662e-04, 2.96160975e-03,
1.58743784e-02, 3.86916241e-03, 7.78142828e-03, 1.89769883e-02,
3.45607921e-02, 5.99975279e-03, 1.32438485e-02, 1.59944184e-02,
4.55442108e-02, 2.63959691e-02, 1.60324126e-02, 7.52872834e-03,
5.19436458e-03, 1.30549502e-02, 2.50185328e-03, 1.31479409e-02,
1.24815470e-02, 3.13224494e-02, 3.86617240e-03, 3.13886553e-02,
6.23510545e-03, 1.51603706e-02, 2.41826810e-02, 2.55665816e-02,
4.22818065e-02, 1.60264131e-02, 9.65361763e-03, 1.14716105e-02,
9.70790097e-06, 1.13144238e-02, 9.17634964e-02, 4.37985696e-02,
3.05680763e-02, 2.89862435e-02, 7.93408509e-03, 6.51481748e-03,
1.63421184e-02, 4.31584604e-02, 1.12092290e-02, 1.97034329e-02,
3.04404330e-02, 6.17427099e-03, 4.72627133e-02, 1.71894114e-02,
1.23116393e-02, 6.64811283e-02, 1.16648125e-02, 5.96572943e-02,
4.68391599e-03, 6.16347231e-03, 3.13578211e-02, 3.49594303e-03,
2.43194420e-02, 2.30796244e-02, 1.13765243e-02, 7.89947510e-02,
1.77858826e-02, 1.54711818e-02, 4.86904988e-03, 5.15150093e-03,
2.23719422e-02, 2.17370000e-02, 1.15967654e-02, 3.13726775e-02,
6.72550453e-03, 1.41271539e-02, 1.65560022e-02, 6.49979152e-03,
6.11034669e-02, 2.93818098e-02, 5.19035794e-02, 7.86356628e-03,
1.95626840e-02, 8.66252556e-03, 3.31655629e-02, 1.28313145e-02,
5.29608969e-03, 2.07320135e-03, 2.65131332e-02, 1.54541889e-02,
1.87976696e-02, 9.98591259e-03, 2.57201474e-02, 1.62325043e-03,
5.17404415e-02, 7.74073377e-02, 5.58550004e-04, 8.15892965e-03,
2.55863238e-02, 9.12082642e-02, 1.07482485e-02, 1.73269007e-02,
5.88654494e-03, 5.83292032e-03, 2.50668135e-02, 1.47695104e-02,
6.34891167e-03, 3.24375741e-02, 3.38039856e-04, 1.36253936e-02,
9.71977599e-03, 1.15634575e-02, 2.09098309e-02, 7.02665560e-03,
8.36278719e-04, 1.65121946e-02, 5.76196052e-02, 3.77912074e-02,
9.13344137e-03, 6.00466225e-03, 2.22463235e-02, 2.18154285e-02,
3.75396572e-02, 7.82074127e-03, 6.74623204e-03, 1.60678010e-02,
7.93319792e-02, 1.19674783e-02, 2.04393733e-02, 9.44191217e-02,
2.02002190e-02, 9.36766248e-03, 3.81874777e-02, 4.08330746e-02,
6.58961385e-03, 1.17035480e-02, 1.76055226e-02, 6.23508394e-02,
1.01111718e-02, 3.51994229e-03, 2.97198794e-03, 1.81857217e-02,
1.39490142e-02, 1.04892636e-02, 1.50694475e-02, 1.97734050e-02,
2.18709074e-02, 1.42058423e-02, 2.13433243e-02, 1.03702499e-02,
1.31554576e-02, 1.15297791e-02, 1.51769631e-02, 1.56679545e-02,
1.07114293e-01, 4.46736664e-02, 1.73115730e-02, 8.61414149e-03,
1.83040984e-02, 2.99416184e-02, 9.47312079e-03, 3.41110560e-03,
3.13109979e-02, 1.54544739e-02, 1.81151666e-02, 2.68737022e-02,
8.39784101e-04, 4.29053837e-03, 1.81923935e-03, 3.81778926e-02,
2.10022107e-02, 8.49787612e-03, 2.01316234e-02, 8.35893769e-03,
5.82060218e-02, 2.16466859e-02, 2.19109990e-02, 5.04137576e-02,
2.31776033e-02, 3.23948893e-03, 7.77044613e-03, 1.71980374e-02,
1.62537992e-02, 1.81431882e-02, 1.76580008e-02, 2.78757345e-02,
3.36593837e-02, 5.56889782e-03, 2.08416414e-02, 3.72145586e-02,
2.11702827e-02, 4.44766432e-02, 2.03032531e-02, 9.55676567e-03,
2.20208848e-03, 1.05860019e-02, 2.03014743e-02, 2.88480567e-03,
5.29586384e-03, 2.02119928e-02, 6.93131890e-03, 8.27069022e-03,
2.13280059e-02, 3.03953118e-03, 1.91182066e-02, 5.02996426e-03,
1.45431254e-02, 1.25880288e-02, 3.33947386e-03, 5.55941090e-03,
3.40723917e-02, 2.57830564e-02, 6.51472211e-02, 1.61715765e-02,
8.74859747e-03, 1.86692160e-02, 1.00166900e-02, 1.06534027e-01,
5.92978019e-03, 5.53775765e-02, 9.90502443e-03, 6.46169409e-02,
6.93583116e-02, 1.05277644e-02, 2.12627053e-02, 5.63361086e-02,
8.50832276e-03, 1.39521463e-02, 3.52553069e-03, 1.67158861e-02,
5.28689325e-02, 3.50338407e-02, 9.86020360e-03, 2.26703212e-02,
1.57220606e-02, 1.67874936e-02, 6.29348448e-03, 1.23281851e-02,
1.66291557e-02, 9.66885500e-03, 9.79695003e-03, 8.18682835e-03,
3.24012036e-03, 2.77784020e-02, 1.01233721e-02, 2.60186959e-02,
1.83296688e-02, 1.10753458e-02, 8.35639238e-03, 8.04111212e-02,
4.24609520e-02, 2.95877345e-02, 8.44371226e-03, 2.21249294e-02,
7.31657632e-03, 2.95627601e-02, 6.83921808e-03, 1.02765420e-02,
6.03820290e-03, 1.96501482e-02, 2.54521854e-02, 6.30441401e-03,
7.53565365e-03, 1.85965467e-03, 2.78363712e-02, 5.92133030e-04,
2.94028199e-03, 1.59678180e-02, 1.06650894e-03, 5.18228561e-02,
9.08512026e-02, 5.90525148e-03, 1.45536577e-02, 4.06972412e-03,
3.40010412e-03, 2.30120053e-03, 2.36487649e-02, 9.26637277e-03,
1.55424913e-02, 3.87809910e-02, 1.83721613e-02, 8.21913127e-03,
2.68836953e-02, 9.62469261e-03, 4.11386937e-02, 6.62071491e-03,
1.02528194e-02, 1.66301932e-02, 1.04250340e-02, 8.48716423e-02,
1.73225813e-02, 4.45605554e-02, 9.10840556e-03, 1.56162139e-02,
2.30812021e-02, 1.60960276e-02, 1.82468854e-02, 3.52374762e-02,
1.44019974e-02, 8.92858356e-02, 3.62466369e-03, 1.88864339e-02,
4.63649770e-03, 1.03525110e-02, 3.66250309e-03, 2.27975454e-02,
3.71446759e-02, 1.69113260e-02, 2.47420389e-02, 1.44357625e-02,
7.97161460e-03, 1.34460563e-02, 2.01785695e-02, 5.95359271e-03,
6.09663920e-03, 1.99881736e-02, 1.22924782e-02, 4.61984053e-02,
1.65765993e-02, 2.12249011e-02, 1.40788145e-02, 1.18310163e-02,
3.47726718e-02, 1.14778839e-02, 5.78994602e-02, 7.90960807e-03,
1.88817624e-02, 8.43468159e-02, 2.66497117e-03, 3.62244658e-02,
1.00187890e-01, 1.60326529e-02, 4.72343192e-02, 1.44964783e-03,
6.85349200e-03, 1.41363265e-02, 1.45742903e-02, 1.60782989e-02,
1.56855527e-02, 1.94098409e-02, 1.73835773e-02, 4.55557648e-03,
1.02387380e-03, 1.18856411e-02, 1.10204043e-02, 1.76420510e-02,
2.46390030e-02, 6.64121239e-03, 2.82916985e-02, 1.42317750e-02,
2.58238483e-02, 1.34375868e-02, 3.28525156e-02, 6.02029637e-03,
1.16223912e-03, 2.03982461e-02, 1.31737934e-02, 6.25290498e-02,
4.59851362e-02, 1.47249382e-02, 2.59405673e-02, 1.01172449e-02,
5.50776124e-02, 1.94475930e-02, 5.84162166e-03, 1.93008967e-02,
4.77783941e-03, 1.42510403e-02, 3.98847908e-02, 2.33372282e-02,
9.20668431e-03, 2.26432481e-03, 1.24019459e-02, 1.78901907e-02,
2.28443388e-02, 2.30312645e-02, 5.87143674e-02, 1.89313088e-02,
1.54532343e-02, 7.30068609e-02, 3.86903249e-03, 4.47305739e-02,
4.26586792e-02, 1.84929911e-02, 1.93615686e-02, 1.73094645e-02,
2.17866823e-02, 1.26524232e-02, 8.65131617e-03, 1.04926163e-02,
2.00351048e-02, 1.12408608e-01, 6.12638183e-02, 4.02530804e-02,
1.04022557e-02, 1.48503939e-02, 3.20976996e-03, 7.57150678e-03,
2.16181632e-02, 2.40094755e-02, 1.85352769e-02, 2.12586168e-02,
6.24792203e-02, 3.38237397e-02, 4.46733972e-03, 1.87009238e-02,
1.29143829e-02, 1.73953343e-02, 2.48064045e-02, 2.26169638e-02,
1.18394233e-02, 2.82253027e-02, 5.56699932e-03, 1.13322642e-02,
1.55691011e-02, 3.06521505e-02, 1.63932182e-02, 2.77797189e-02,
3.09825782e-02, 7.73158483e-03, 9.05308407e-03, 1.12431431e-02,
3.71876848e-03, 6.67216349e-03, 1.18998773e-02, 1.62736829e-02,
9.82116815e-03, 4.60728956e-03, 8.04538745e-03, 4.72667394e-03,
2.29740106e-02, 2.96942168e-03, 9.27346125e-02, 2.36750790e-03,
3.88836786e-02, 4.15088385e-02, 4.69338754e-03, 1.90786459e-02,
1.46014486e-02, 5.24344062e-03, 2.36579031e-02, 1.74694862e-02,
9.03700385e-03, 1.05457939e-01, 6.84238672e-02, 6.42049266e-03,
2.89540570e-02, 4.05897433e-03, 1.25274304e-02, 2.88230088e-02,
7.51970187e-02, 9.08205006e-03, 1.64980423e-02, 4.84195072e-03,
2.82078534e-02, 1.42947817e-02, 1.83642674e-02, 5.83109856e-02,
1.16234142e-02, 1.11154113e-02, 1.25350300e-02, 1.74971968e-02,
4.24703732e-02, 2.08942872e-02, 6.67405734e-03, 1.45699391e-02,
7.57706398e-03, 1.39211183e-02, 2.70018429e-02, 1.88221447e-02,
9.12061241e-03, 1.54390186e-02, 1.84007362e-02, 2.77795792e-02,
4.74580005e-02, 6.66268449e-03, 1.32328533e-02, 2.49137320e-02,
8.38182028e-03, 1.44902011e-02, 3.56387310e-02, 1.73001811e-02,
6.13669120e-03, 1.21898539e-02, 4.93628345e-02, 4.39124741e-03,
6.81095431e-03, 8.11346024e-02, 5.14589921e-02, 7.81604182e-03,
5.29955178e-02, 1.77882798e-02, 1.53298704e-02, 1.38059566e-02,
2.59595551e-02, 2.93541905e-02, 1.10675450e-02, 7.37368912e-02,
1.29241757e-02, 4.72766235e-02, 1.15967259e-01, 8.40159804e-02,
1.96566563e-02, 2.98437150e-03, 2.42527407e-02, 3.37757990e-02,
1.61876734e-02, 2.26045912e-03, 4.03943025e-02, 1.46772778e-02,
1.55659346e-03, 2.66074240e-02, 1.59585022e-03, 3.75781134e-02,
3.90417054e-02, 1.11415423e-02, 6.05571782e-03, 7.12481933e-03,
1.20715853e-02, 5.92063507e-03, 2.14886032e-02, 1.38464291e-02,
3.63497902e-03, 3.43065034e-03, 1.85409728e-02, 2.00014543e-02,
1.72978155e-02, 1.22506088e-02, 2.77608912e-02, 4.96756565e-03,
3.58079153e-04, 1.93737317e-02, 1.64496582e-02, 2.99644121e-03,
9.17024072e-03, 3.02798860e-02, 2.93213539e-02, 2.31711864e-02,
2.55345926e-02, 5.49242925e-03, 2.05399450e-02, 2.55491659e-02,
8.88210535e-02, 1.35292942e-02, 5.19566089e-02, 9.52861179e-03,
1.22125139e-02, 5.97844599e-03, 1.30045926e-02, 2.30349824e-02,
1.77120119e-02, 2.14994196e-02, 2.81897280e-02, 9.97961778e-03,
6.78729862e-02, 3.19206975e-02, 1.71383079e-02, 1.98331438e-02,
1.34726148e-02, 4.79090400e-03, 5.40045537e-02, 2.03534327e-02,
6.82575814e-03, 6.73405722e-08, 2.01796480e-02, 8.61796457e-03,
6.33951928e-03, 2.25231852e-02, 9.29878838e-03, 1.36235459e-02,
3.43488716e-02, 1.93990972e-02, 1.33903269e-02, 2.37084157e-03,
2.82562952e-02, 1.60772018e-02, 2.36852448e-02, 1.13479495e-02,
2.62321830e-02, 2.33993810e-02, 1.62304919e-02, 8.99812672e-03,
2.47323401e-02, 8.23134184e-03, 9.60064121e-03, 7.28827436e-03,
1.03339911e-01, 2.04712562e-02, 3.74096744e-02, 4.27896343e-02,
5.69646852e-03, 4.08471897e-02, 1.57504398e-02, 8.35492928e-03,
5.28718997e-03, 9.18967873e-02, 1.56131247e-02, 1.52842915e-02,
2.88751889e-02, 5.19211367e-02, 2.26678443e-03, 5.42339385e-02,
2.49185301e-02, 3.47074494e-02, 1.21471975e-02, 8.05945043e-03,
4.80777249e-02, 1.33638857e-02, 2.52536088e-02, 2.96427924e-02,
1.89067852e-02, 2.41315532e-02, 2.76871352e-03, 1.64503530e-02,
1.27208140e-02, 4.21547331e-03, 3.46949860e-03, 3.80067453e-02,
1.99430287e-02, 6.36423705e-03, 1.24060316e-02, 1.96298901e-02,
4.74744476e-03, 1.15611665e-02, 9.36791301e-03, 5.76191163e-03,
5.38968900e-03, 1.10690948e-02, 1.31403981e-02, 3.14705037e-02,
1.87346172e-02, 1.29758529e-02, 6.32049469e-03, 2.71914545e-02,
5.25918975e-02, 1.96794365e-02, 2.50179265e-02, 2.33531352e-02,
3.28052156e-02, 1.57408752e-02, 8.03319551e-03, 6.69207284e-03,
9.77990124e-03, 6.37487229e-03, 1.79526315e-03, 2.53329054e-02,
3.95053923e-02, 7.35301599e-02, 5.89747727e-02, 3.02571617e-02,
2.10695788e-02, 5.64318895e-02, 3.41955312e-02, 9.31384694e-03,
2.63935085e-02, 9.19133890e-03, 3.17199482e-03, 5.06662996e-03,
1.22588426e-02, 2.09351368e-02, 2.44578086e-02, 1.27928769e-02], dtype=float32), age=Array([ 6.2660956 , 7.5452933 , 6.0236206 , 8.38898 , 6.143864 ,
11.029141 , 6.2312727 , 10.923996 , 7.448931 , 6.4165735 ,
10.558735 , 6.334644 , 6.2014194 , 4.6322618 , 3.2757847 ,
11.221254 , 10.3444195 , 10.089584 , 8.365385 , 0.2600517 ,
10.578849 , 10.510507 , 7.731289 , 9.048802 , 9.3716755 ,
8.268257 , 12.117719 , 9.172806 , 10.678836 , 8.343397 ,
7.4854493 , 12.663981 , 6.4712434 , 9.830652 , 6.653867 ,
11.864302 , 5.3480453 , 7.368439 , 9.723574 , 5.6775823 ,
9.963281 , 4.619538 , 6.626815 , 4.3986936 , 9.4302 ,
5.403395 , 6.7577815 , 7.4006143 , 11.829933 , 7.455671 ,
10.906418 , 6.580483 , 5.146871 , 10.560664 , 10.843436 ,
10.475463 , 9.5986595 , 6.318466 , 11.309216 , 6.03153 ,
8.827878 , 10.193798 , 6.6616306 , 6.7273626 , 7.019449 ,
9.90719 , 9.11537 , 9.865308 , 11.273972 , 7.986376 ,
4.929704 , 11.532095 , 11.960674 , 2.860077 , 10.097944 ,
6.76441 , 7.2991505 , 6.914495 , 9.945149 , 12.022414 ,
1.8150936 , 9.920713 , 9.350282 , 1.9598546 , 10.7600565 ,
12.272347 , 6.8989244 , 9.726943 , 10.73986 , 6.488827 ,
5.409971 , 6.41422 , 8.009001 , 10.766486 , 6.475249 ,
11.028538 , 7.6853843 , 7.85695 , 3.6750443 , 6.5161953 ,
8.734444 , 7.583246 , 6.9275146 , 10.169469 , 6.575232 ,
11.654101 , 4.9744925 , 5.4023986 , 10.085197 , 7.1551642 ,
9.497396 , 6.203664 , 5.518049 , 8.841917 , 10.785697 ,
8.430336 , 4.7108893 , 9.871827 , 6.1664147 , 7.482253 ,
8.589218 , 10.2719555 , 6.1585627 , 9.251525 , 9.4826355 ,
7.2670107 , 4.4895425 , 7.0912786 , 5.0599027 , 5.852671 ,
3.491022 , 8.822149 , 9.582982 , 7.2508917 , 11.473682 ,
10.172787 , 10.315979 , 9.914061 , 6.4022574 , 10.063596 ,
10.522583 , 8.802703 , 6.0616064 , 9.545591 , 11.589317 ,
5.9453287 , 0.9081424 , 10.903268 , 6.633187 , 6.681497 ,
9.813433 , 10.1274605 , 6.38156 , 4.831305 , 11.383838 ,
10.432071 , 6.783573 , 8.995797 , 7.6751084 , 10.73088 ,
8.990998 , 9.673606 , 10.56084 , 4.5774603 , 4.4757733 ,
8.629637 , 4.5825143 , 10.693021 , 6.5025654 , 6.347055 ,
6.2731743 , 2.4580543 , 7.5492563 , 10.953272 , 5.9836974 ,
3.2422793 , 11.014155 , 1.5037681 , 6.3708296 , 5.865255 ,
4.599258 , 6.962362 , 5.8970037 , 6.28236 , 5.3468437 ,
9.918635 , 7.527518 , 9.811515 , 6.831124 , 5.3432374 ,
5.2013326 , 12.005889 , 11.544124 , 9.255129 , 8.953854 ,
6.2906194 , 7.760603 , 5.8780136 , 4.122131 , 6.6218557 ,
6.0198507 , 9.72303 , 7.5281534 , 8.981387 , 10.250799 ,
7.228799 , 7.281129 , 6.031906 , 5.7078977 , 8.525396 ,
8.1356 , 1.8639915 , 4.9415627 , 6.501848 , 6.2457514 ,
9.8756075 , 6.3575373 , 7.53387 , 5.375653 , 3.944124 ,
9.21607 , 9.676683 , 6.2950206 , 6.105249 , 7.2167377 ,
6.5706906 , 11.330916 , 8.692481 , 10.850498 , 7.263888 ,
9.971086 , 9.80597 , 9.314813 , 7.451499 , 7.652406 ,
10.5896435 , 2.095729 , 4.2417397 , 1.8585155 , 8.739205 ,
7.6981297 , 9.393969 , 10.120547 , 6.3507476 , 5.5103483 ,
10.166539 , 7.3066745 , 9.656752 , 6.8737707 , 11.137563 ,
9.739094 , 7.3604455 , 7.126126 , 9.371441 , 6.714702 ,
10.25462 , 7.493115 , 6.2759304 , 10.922744 , 0.2983044 ,
7.0316334 , 11.249139 , 6.4337626 , 10.030162 , 7.3651543 ,
11.059778 , 9.714322 , 7.5383153 , 7.221201 , 8.479709 ,
2.8850114 , 4.228602 , 10.21122 , 7.8551273 , 9.671846 ,
9.872667 , 4.3746343 , 12.932107 , 6.721057 , 6.3327255 ,
11.007318 , 9.670086 , 4.643705 , 10.448043 , 3.0407183 ,
7.2525373 , 10.693694 , 6.3146696 , 2.8858383 , 9.376599 ,
7.3555727 , 6.7015843 , 6.3710117 , 9.972727 , 5.510028 ,
10.622855 , 10.1708355 , 6.8938994 , 6.517987 , 6.9021435 ,
6.490849 , 7.1244535 , 10.873499 , 9.207714 , 9.438536 ,
9.673606 , 11.215472 , 8.924899 , 7.215912 , 11.88843 ,
6.7605734 , 5.073228 , 8.280675 , 6.609268 , 6.5697994 ,
4.7231064 , 10.43498 , 10.155974 , 6.558211 , 6.5534825 ,
6.76319 , 1.9278631 , 7.315826 , 10.434616 , 0.66946083,
8.094644 , 11.38965 , 9.942671 , 10.69066 , 4.5311956 ,
6.4009423 , 7.25122 , 7.51543 , 5.990692 , 5.411566 ,
7.542757 , 7.528788 , 11.769681 , 6.644681 , 4.704487 ,
3.895182 , 9.891952 , 8.121001 , 5.9061527 , 7.5179763 ,
7.102184 , 11.856621 , 11.854482 , 9.6852455 , 8.445633 ,
10.802675 , 7.4511786 , 4.1526456 , 7.435593 , 7.152248 ,
6.574052 , 3.7355504 , 8.546199 , 6.3358307 , 7.569036 ,
6.58582 , 8.857474 , 8.693014 , 6.3049192 , 10.805767 ,
5.699161 , 7.779976 , 10.238163 , 8.153657 , 7.4106245 ,
7.061341 , 9.755963 , 3.6161277 , 4.7586417 , 6.3279743 ,
10.760386 , 13.043479 , 6.4590373 , 6.32569 , 4.4504495 ,
7.264381 , 9.290548 , 7.9478016 , 11.648439 , 5.0421486 ,
4.519446 , 7.7416396 , 8.536354 , 7.5964837 , 10.126474 ,
8.672188 , 8.254669 , 8.111642 , 1.1785314 , 10.72738 ,
6.656779 , 9.316711 , 6.5777693 , 9.174758 , 11.0522995 ,
5.849511 , 5.664927 , 8.33718 , 0.3702414 , 6.181219 ,
9.701229 , 10.695379 , 10.444781 , 5.829284 , 9.444548 ,
8.228557 , 8.819609 , 6.2365317 , 6.805124 , 7.540219 ,
9.84273 , 10.4697075 , 4.197971 , 2.2229176 , 10.626296 ,
6.797483 , 7.097572 , 10.108865 , 6.2188063 , 11.145686 ,
11.753551 , 6.03567 , 6.448168 , 9.849917 , 10.79485 ,
7.632611 , 11.259711 , 6.7070265 , 4.025125 , 11.477249 ,
10.736869 , 9.503791 , 4.3334327 , 7.7127132 , 11.215472 ,
10.722208 , 11.15898 , 10.355585 , 8.446467 , 6.421417 ,
7.5068307 , 11.976806 , 9.821945 , 6.742104 , 9.894878 ,
4.721001 , 10.428794 , 12.732711 , 10.3095875 , 2.0809479 ,
7.313213 , 8.392764 , 11.75939 , 7.4354324 , 5.9340286 ,
9.370502 , 10.143022 , 6.072862 , 6.368828 , 3.4285738 ,
11.110994 , 7.8502636 , 6.0754867 , 9.7064705 , 8.096404 ,
5.3956175 , 9.728029 , 9.972111 , 6.889775 , 7.673551 ,
6.078626 , 6.4860415 , 10.783731 , 10.64584 , 11.679398 ,
10.979191 , 10.528252 , 6.2944717 , 6.138765 , 6.340212 ,
7.526563 , 5.815074 , 9.563423 , 6.2892427 , 9.878336 ,
6.334917 , 6.9895945 , 5.625907 , 4.636925 , 6.933674 ,
10.377723 , 6.017589 , 5.606523 , 6.1082845 , 10.984715 ,
5.3969145 , 10.933682 , 6.1894712 , 5.6723266 , 9.989511 ,
11.06515 , 11.88464 , 5.7109056 , 6.2762976 , 8.246072 ,
8.483783 , 7.1914015 , 1.5494807 , 6.496642 , 6.120843 ,
2.6185892 , 9.694229 , 8.819021 , 6.481366 , 7.49886 ,
5.971681 , 6.740487 , 6.9823265 , 4.73342 , 5.4183373 ,
7.4998164 , 6.272439 , 4.6817746 , 6.294287 , 8.598976 ,
7.7736783 , 6.421462 , 11.704403 , 6.2483306 , 6.2985964 ,
6.8356237 , 6.998427 , 6.312793 , 6.823289 , 6.732965 ,
7.8951244 , 10.790277 , 6.379106 , 7.2867036 , 5.757271 ,
8.885882 , 7.707132 , 10.700089 , 8.010189 , 9.184021 ,
1.8116634 , 6.2725315 , 10.391915 , 7.9887614 , 6.5222855 ,
4.7533913 , 6.6025243 , 0.7417018 , 7.2591195 , 11.458981 ,
4.643598 , 12.425718 , 9.855405 , 6.097396 , 6.496956 ,
11.767448 , 6.606075 , 9.79379 , 2.1925395 , 5.5192337 ,
6.4044337 , 6.583864 , 6.1457253 , 6.868085 , 7.0889273 ,
5.5592265 , 6.0729556 , 6.451641 , 11.079576 , 10.4711485 ,
10.732211 , 6.303636 , 9.924035 , 6.1509137 , 9.0212345 ,
6.9199796 , 10.848253 , 3.5597887 , 4.4633327 , 6.621147 ,
8.429221 , 8.603308 , 11.079428 , 5.7642236 , 8.670583 ,
9.074984 , 11.840091 , 10.840864 , 8.842956 , 9.237084 ,
11.001074 , 11.872377 , 5.650939 , 11.794084 , 10.3444195 ,
10.882397 , 13.044149 , 3.1404426 , 5.654011 , 9.3535795 ,
11.047503 , 9.462075 , 11.1197815 , 11.19165 , 6.3571734 ,
10.7230425 , 7.1926875 , 6.2673373 , 7.345817 , 6.6096234 ,
4.4534564 , 6.978712 , 4.6555076 , 10.114216 , 10.570468 ,
7.3367014 , 8.134652 , 4.336059 , 7.2491627 , 5.955906 ,
10.525773 , 10.3232975 , 5.5749483 , 6.4209194 , 6.3829226 ,
7.3870325 , 8.41081 , 6.087406 , 11.393868 , 6.123735 ,
6.546386 , 6.6672745 , 8.016129 , 6.300636 , 1.7231808 ,
7.644862 , 9.774488 , 8.613042 , 6.9702 , 7.2238445 ,
7.0106406 , 9.402308 , 11.078537 , 7.540377 , 10.475103 ,
1.9524193 , 9.061285 , 10.05677 , 4.9597173 , 10.082054 ,
7.3607707 , 7.960833 , 0.46186903, 7.1641555 , 6.7350655 ,
9.843787 , 10.933994 , 9.495568 , 5.4789505 , 7.816735 ,
8.414162 , 11.466023 , 10.716859 , 6.93641 , 6.2940125 ,
6.8245454 , 5.204577 , 10.014763 , 6.6240697 , 9.387367 ,
12.95674 , 7.219548 , 7.1531653 , 7.0319715 , 7.7383966 ,
8.809628 , 6.270325 , 10.312221 , 9.544629 , 9.971906 ,
6.7473035 , 12.478151 , 11.642763 , 8.46311 , 6.2609444 ,
6.632856 , 6.144609 , 10.601097 , 7.687795 , 8.60439 ,
3.0997772 , 6.379037 , 6.8383913 , 10.665949 , 10.56732 ,
10.041875 , 3.827223 , 6.8013043 , 8.4492445 , 11.778144 ,
11.514373 , 6.2398515 , 6.257608 , 5.95785 , 1.8871257 ,
9.631185 , 8.778203 , 4.230783 , 8.576453 , 8.351017 ,
4.45002 , 6.6152987 , 9.724335 , 7.055694 , 7.906446 ,
8.798126 , 9.012685 , 9.9678 , 6.138789 , 6.293554 ,
4.177405 , 8.725838 , 8.497915 , 6.358631 , 9.786509 ,
9.555079 , 7.6473255 , 9.534269 , 9.811302 , 10.16693 ,
0.7252882 , 5.5592265 , 10.682557 , 8.88627 , 7.002839 ,
5.955574 , 7.7358093 , 6.1358542 , 7.6749535 , 5.813536 ,
12.263724 , 6.450695 , 6.590443 , 5.0203567 , 6.813799 ,
5.6567407 , 9.13557 , 6.9861956 , 8.964148 , 11.00336 ,
11.725932 , 10.187195 , 10.12608 , 6.2276263 , 7.6663795 ,
10.998176 , 7.7096906 , 8.943284 , 9.595528 , 9.422314 ,
6.316064 , 10.756755 , 6.5530357 , 2.428507 , 8.35017 ,
6.3553505 , 8.780303 , 11.029293 , 5.5409217 , 8.119832 ,
9.235396 , 3.9204803 , 3.943401 , 10.890003 , 9.486874 ,
9.625854 , 6.3436794 , 6.5728283 , 4.8726387 , 10.516195 ,
6.4672365 , 7.3867083 , 5.8789682 , 10.35075 , 7.4115925 ,
1.9318333 , 9.98256 , 10.673077 , 5.404441 , 6.8363156 ,
5.3706555 , 6.439907 , 10.020443 , 6.9997845 , 6.6866446 ,
9.6586275 , 7.5036435 , 6.28011 , 6.6147666 , 7.510336 ,
10.645328 , 5.4021993 , 3.3578272 , 11.699564 , 6.4914327 ,
7.124119 , 10.197676 , 6.4781275 , 5.440216 , 6.3061333 ,
8.635695 , 6.978031 , 3.7450106 , 11.789668 , 10.89032 ,
5.5832915 , 1.9786798 , 6.9878955 , 1.0501271 , 8.146673 ,
9.584328 , 5.916056 , 8.177464 , 5.447169 , 7.471055 ,
5.7094502 , 8.628155 , 3.3123481 , 9.107528 , 4.081971 ,
10.894747 , 12.527755 , 6.2282267 , 4.6610613 , 6.3666434 ,
10.076011 , 6.158191 , 6.3366513 , 11.326591 , 6.1443295 ,
11.648911 , 1.5290347 , 9.379879 , 7.619751 , 10.270625 ,
10.438251 , 10.999396 , 9.2387705 , 5.209745 , 6.4561515 ,
10.7375345 , 10.906418 , 6.2897935 , 9.964514 , 6.238007 ,
8.949399 , 5.8104606 , 7.008267 , 13.483831 , 7.485928 ,
6.2709684 , 10.117383 , 6.659867 , 6.711238 , 5.541907 ,
9.562747 , 5.1607122 , 10.105494 , 4.5585036 , 9.614728 ,
4.451739 , 7.2957134 , 6.4368806 , 8.1994505 , 7.543708 ,
10.398992 , 8.35137 , 5.6766095 , 6.220839 , 7.0377183 ,
5.0704613 , 6.5120726 , 1.3788162 , 9.474721 , 6.2019744 ,
6.5110865 , 7.7023215 , 8.410252 , 2.097206 , 8.638387 ,
11.422154 , 13.072409 , 7.219548 , 10.030365 , 9.007649 ,
11.638852 , 6.6375875 , 5.5032363 , 8.226255 , 7.5751185 ,
6.6039443 , 9.609378 , 6.574876 , 6.324502 , 11.548202 ,
6.5272985 , 6.04075 , 6.3193808 , 6.444648 , 6.594443 ,
5.841559 , 10.433162 , 11.910807 , 11.59053 , 5.867214 ,
8.554392 , 4.3684783 , 4.264718 , 11.636419 , 4.367721 ,
9.949278 , 10.23471 , 9.00513 , 6.617514 , 5.044818 ,
6.56374 , 5.9834123 , 3.5904546 , 11.3228 , 7.0224977 ,
6.482805 , 4.836316 , 7.1764603 , 7.464969 , 6.149914 ,
10.794198 , 8.284809 , 6.2515545 , 10.667138 , 6.75501 ,
11.453717 , 9.969853 , 10.0127325 , 10.170054 , 10.493387 ,
4.8003626 , 8.3354845 , 6.730339 , 10.137119 , 5.8983393 ,
6.7651076 , 6.335283 , 6.3443866 , 8.822149 , 7.7916365 ,
10.2301 , 6.1745396 , 5.74316 , 6.153449 , 9.903646 ,
7.9665174 , 4.52647 , 3.4685907 , 6.275379 , 9.25705 ,
6.6490984 , 5.6148434 , 9.808211 , 9.210986 , 11.577889 ,
10.392145 , 8.682878 , 11.49146 , 9.186821 , 4.2905927 ,
5.9688873 , 0.7820431 , 3.319744 , 6.399899 , 2.0419753 ,
4.984504 , 10.069207 , 5.433458 , 7.972048 , 9.592842 ,
6.690865 , 7.133813 , 6.3338213 , 7.44604 , 7.5983734 ], dtype=float32), pixel_assignment=None, spatial_bin_edges=None, mask=None, spectra=None, datacube=None), gas=GasData(coords=None, velocity=None, mass=None, density=None, internal_energy=None, metallicity=None, sfr=None, electron_abundance=None, pixel_assignment=None, spatial_bin_edges=None, mask=None, spectra=None, datacube=None))
[4700.15 4701.4 4702.65 ... 9347.65 9348.9 9350.15]
[1645.0009 1643.6793 1642.358 ... 128.56454 128.44652 128.32852]
[<matplotlib.lines.Line2D at 0x7f606831f040>]
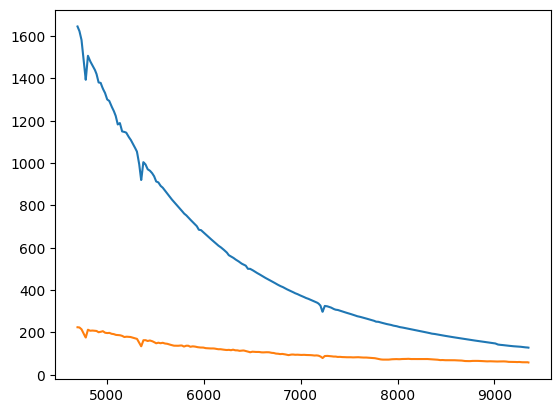
Step 10: Datacube#
Now we can add all stellar spectra that contribute to one spaxel and get the IFU datacube. The plot shows the spatial dimension of the datacube
, where we summed over the wavelength dimension.
# NBVAL_SKIP
from rubix.core.ifu import get_calculate_datacube
calculate_datacube = get_calculate_datacube(config)
rubixdata = calculate_datacube(rubixdata)
/home/annalena/rubix/rubix/telescope/factory.py:24: UserWarning: No telescope config provided, using default stored in /home/annalena/rubix/rubix/telescope/telescopes.yaml
warnings.warn(
2024-12-04 12:39:42,065 - rubix - INFO - Calculating Data Cube...
2024-12-04 12:39:42,131 - rubix - DEBUG - Datacube Shape: (25, 25, 3721)
# NBVAL_SKIP
datacube = rubixdata.stars.datacube
img = datacube.sum(axis=2)
plt.imshow(img, origin="lower")
<matplotlib.image.AxesImage at 0x7f606812d600>
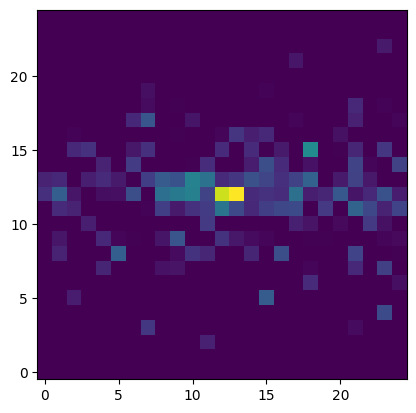
Step 11: PSF#
The instrument and the earth athmosphere affect the spatial resolution of the observation data and smooth in spatial dimention. To take this effect into account we convolve our datacube with a point spread function (PSF).
# NBVAL_SKIP
from rubix.core.psf import get_convolve_psf
convolve_psf = get_convolve_psf(config)
rubixdata = convolve_psf(rubixdata)
2024-12-04 12:39:42,443 - rubix - INFO - Convolving with PSF...
# NBVAL_SKIP
datacube = rubixdata.stars.datacube
img = datacube.sum(axis=2)
plt.imshow(img, origin="lower")
<matplotlib.image.AxesImage at 0x7f604871dcf0>
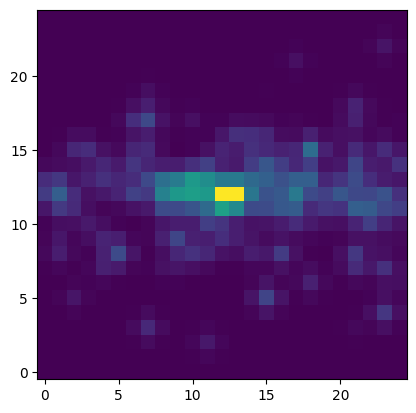
# NBVAL_SKIP
plt.plot(wave, datacube[12,12,:])
plt.plot(wave, datacube[0,0,:])
[<matplotlib.lines.Line2D at 0x7f60487e05b0>]
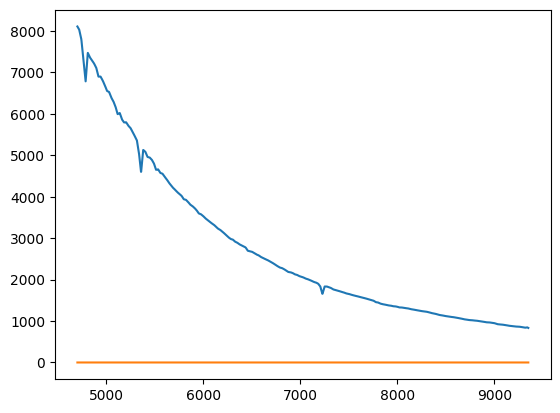
Step 11: LSF#
The instrument affects the spectral resolution of the observation data and smooth in spectral dimention. To take this effect into account we convolve our datacube with a line spread function (LSF).
# NBVAL_SKIP
from rubix.core.lsf import get_convolve_lsf
convolve_lsf = get_convolve_lsf(config)
rubixdata = convolve_lsf(rubixdata)
plt.plot(wave, rubixdata.stars.datacube[12,12,:])
plt.plot(wave, rubixdata.stars.datacube[0,0,:])
/home/annalena/rubix/rubix/telescope/factory.py:24: UserWarning: No telescope config provided, using default stored in /home/annalena/rubix/rubix/telescope/telescopes.yaml
warnings.warn(
2024-12-04 12:39:42,904 - rubix - INFO - Convolving with LSF...
[<matplotlib.lines.Line2D at 0x7f60486da1d0>]
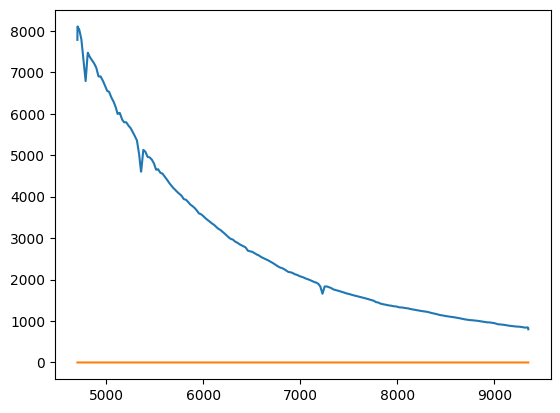
Step 12: Noise#
Observational data are never noise-free. We apply noise to our mock-datacube to mimic real measurements.
# NBVAL_SKIP
from rubix.core.noise import get_apply_noise
apply_noise = get_apply_noise(config)
rubixdata = apply_noise(rubixdata)
datacube = rubixdata.stars.datacube
img = datacube.sum(axis=2)
plt.imshow(img, origin="lower")
2024-12-04 12:39:43,355 - rubix - INFO - Applying noise to datacube with signal to noise ratio: 1 and noise distribution: normal
<matplotlib.image.AxesImage at 0x7f60483e7ac0>
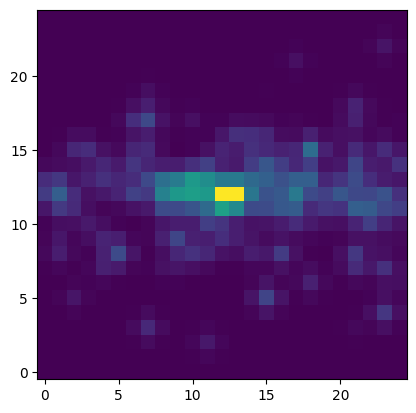
# NBVAL_SKIP
plt.plot(wave, rubixdata.stars.datacube[12,12,:])
plt.plot(wave, rubixdata.stars.datacube[0,0,:])
[<matplotlib.lines.Line2D at 0x7f60484a43d0>]
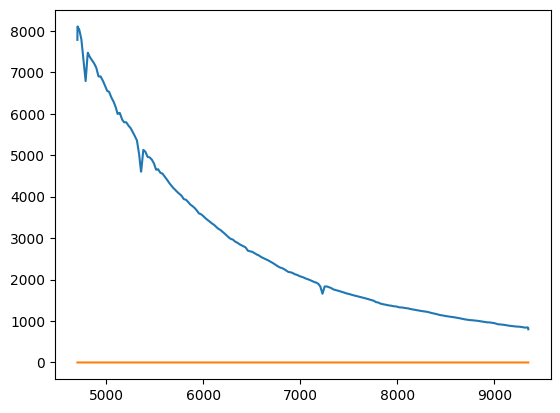
DONE!#
Congratulations, you have now created step by step your own mock-observed IFU datacube! Now enjoy playing around with the RUBIX pipeline and enjoy doing amazing science with RUBIX :)